In this section we are going to review searching and sorting algorithms.
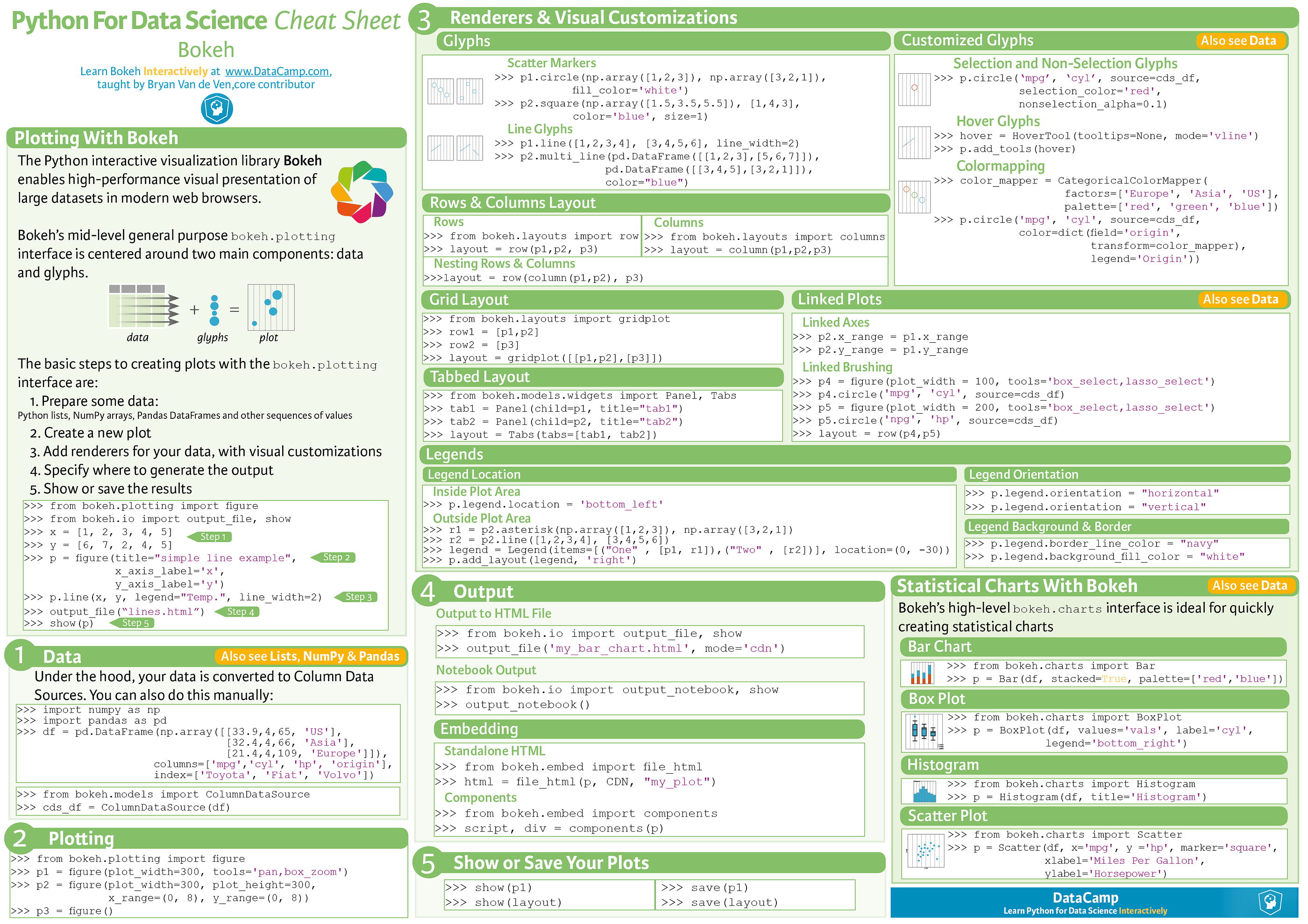
Big O notation is mostly used, and it is used mainly all the times to find the upper bound of an algorithm whereas the Big θ notation is sometimes used which is used to detect the average case and the Ω notation is the least used notation among the three. If the array is sorted, you can use a binary search instead. This will be much more efficient, since binary search runs in worst-case logarithmic time, making O(log n) comparisons, where n is the size of the slice. There are the three custom binary search functions: sort.SearchInts, sort.SearchStrings or sort.SearchFloat64s. They all have the.
Why is sorting so important
- The first step in organizing data is sorting. Lots of tasks become easier once a data set of items is sorted
- Some algorithms like binary search are built around a sorted data structure.
- In accordance to S. Skiena computers have historically spent more time sorting than doing anything else. Sorting remains the most ubiquitous combinatorial algorithm problem in practice.
Considerations:
- How to sort: descending order or ascending order?
- Sorting based on what? An object name (alphabetically), by some number defined by its fields/instance variables. Or maybe compare dates, birthdays, etc.
- What happens with equals keys, for example various people with the same name: John, then sort them by Last Name.
- Does your sorting algorithm sorts in place or needs extra memory to hold another copy of the array to be sorted. This is even more important in embedded systems.
Java uses Comparable interface for sorting and returns: +1 if compared object is greater, -1 if compared object is less and 0 if compared objects are equal.
Sorting becomes more ubiquitous when we think on all the things we do daily that are previously sorted for us to understand and have better access to them:
- Imagine trying to find a phone number in an unsorted phone book, or searching for a word in an unsorted dictionary.
- Your MP3 player can sort your lists by artists name, genre, song name, ratings.
- Search engines display results in descending order of importance
- Spreadsheets can be sorted in various ways to work better with their contents
What Is The Big-o Notation For The `len()` Function In Python?
Searching
Python Big O Notation
There are two types of searching algorithms: Those that need a previously ordered data structure in order to work properly, and those that don’t need an ordered list.

Python - How Do I Calculate Time Complexity For This ...
Searching is also very important for many computing applications: searching through a search engine, finding a bank account balance for some client, searching in a large data set for a particular value, searching in your directories for some needed file. Many applications rely on effective search, if your application is complete but takes long too perform a search and retrieve data it will be discarded as useless.
